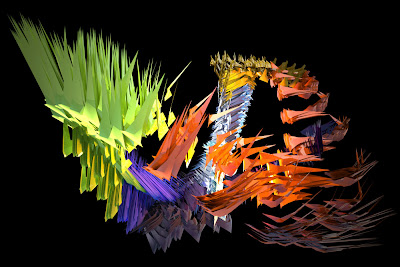
Recently finished Nick Pisca's MEL scripting workshop and found MEL is a lot of fun. Seems much smarter than Rhino in a lot of ways. Things like declarations and arrays are much easier although the case sensitive stuff gets me all the time. We didn't cover procedures in the workshop but got to hit a lot of ground including scripting material assignment and rendering.
Here's a simple tracking script where planes duplicate and deform while following an animated object. I'll post the video sometime in the future.
//Tracking script
//
//Written by: Sky Milner
//Date: 3-03-08
//Contributor: Nick Pisca
//
//This script duplicates selected objects based on the
//location of a target.
//
//Setup:
//First make an object and call it "Target", Animate target as you like, make 6 nurbsPlanes
//
//
//++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
//++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
//++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
string $Selection[] = {"nurbsPlane1", "nurbsPlane2", "nurbsPlane2", "nurbsPlane3", "nurbsPlane3","nurbsPlane4","nurbsPlane4","nurbsPlane5","nurbsPlane5","nurbsPlane6", "nurbsPlane6","nurbsPlane1"};
int $SelSize = size($Selection);
int $SquareCounter = 0;
for ($i = 0; $i < currentnumber =" 0;" selectioncounter =" 0;" firstobject =" $Selection[$CurrentNumber];" secondobject =" $Selection[$CurrentNumber+1];" vecfirst =" <<$XYZfirst[0],">> ;
float $XYZsecond[] = `pointPosition ($SecondObject + ".cv [0] [0]")` ;
vector $VecSecond = <<$XYZsecond[0], $XYZsecond[1], $XYZsecond[2]>> ;
float $XYZtarget[] = `pointPosition ("Target.cv [0] [0]")`;
vector $VecTarget = <<$XYZtarget[0], $XYZtarget[1], $XYZtarget[2]>>;
$Between = $VecFirst - $VecSecond;
//Duplicate and move into position
duplicate -rr -n ("series"+$i+"square"+$SquareCounter) $Selection[($CurrentNumber)];
string $Duplicate = ("series"+$i+"square"+$SquareCounter);
//setAttr ($Duplicate+ ".translate") ($XYZfirst[0] + $Between.x/(-2)) ($XYZfirst[1] + $Between.y / (-2) ) ($XYZfirst[2] + $Between.z / (-2));
xform -r -t ($Between.x/(-2)) ($Between.y / (-2)) ($Between.z / (-2)) $Duplicate;
float $XYZduplicate[] = `pointPosition ($Duplicate + ".cv [0] [0]")`;
vector $Vecduplicate = <<$XYZduplicate[0], $XYZduplicate[1], $XYZduplicate[2]>>;
$Between2 = $VecTarget - $Vecduplicate;
//setAttr ($Duplicate+ ".translate") ($XYZduplicate[0] + $Between2.x/4) ($XYZduplicate[1] + $Between2.y/2) ($XYZduplicate[2] + $Between2.z/4);
xform -r -t ($Between2.x/4) ($Between2.y/2) ($Between2.z/4) $Duplicate;
//Scale Duplicate
float $scalefactor = ((mag($Between2)*.01)+.75);
//setAttr ($Duplicate+ ".scaleX") $scalefactor;
//setAttr ($Duplicate+ ".scaleY") $scalefactor;
//setAttr ($Duplicate+ ".scaleZ") $scalefactor;
//Manipulate cv[0]
float $PointX = `getAttr ($Duplicate + ".cv[0].xValue")`;
float $PointY = `getAttr ($Duplicate + ".cv[0].yValue")`;
float $PointZ = `getAttr ($Duplicate + ".cv[0].zValue")`;
vector $VecPoint = <<$PointX, $PointY, $PointZ>>;
$Between3 = $VecTarget - $VecPoint;
setAttr ($Duplicate+ ".cv[0].xValue") ($PointX + $Between3.x/(10));
setAttr ($Duplicate+ ".cv[0].yValue") ($PointY + $Between3.y/(10));
setAttr ($Duplicate+ ".cv[0].zValue") ($PointZ + $Between3.z/(10));
//setAttr ($Duplicate + ".objectColor") $SquareCounter;
$TempSelection [$SelectionCounter] = $FirstObject;
$TempSelection [$SelectionCounter+1] = $Duplicate;
$TempSelection [$SelectionCounter+2] = $SecondObject;
$TempSelection [$SelectionCounter+3] = $Duplicate;
$CurrentNumber = $CurrentNumber+2;
$SelectionCounter = $SelectionCounter + 4;
$SquareCounter++;
} while ($CurrentNumber < $SelSize); currentTime $i; $Selection = $TempSelection; $SelSize = size($Selection); if ($SelSize > 100) {
for ($s = 0; $s < selsize =" 100;" iii =" 0;" sizejump =" size($jump);" nicklambert = "nicklambert" nicklambertsg = "nicklambert" nicklambertoutcolor = "nicklambert" nicklambertsgss = "nicklambert" nicklamberttrans = "nicklambert" iiiend =" $iii" iiiend2 =" $iii" iii="$iii" i="0;" seriessize =" size($series);" s =" 0;" curshader =" $Shader[0];">